C++ has a set of rich and powerful control structures (statements) that makes it a popular language. Control structure generally fall into four categories but in this unit we have to know only three of them, which are:
i. sequence structure
ii. selection structure
iii. repetition or iteration structure
a-Sequence structureThe sequence control structure is the simplest of all the structures. The program instructions are executed one by one, starting from the first instruction and ending in the last instruction as in the program segment.
b-Selection StructureThe selection structure allows to be executed non-sequentially. It allows the comparison of two expressions, and based on the comparison, to select a certain course of action. In C++ , there are three types of selection statements. They are:
i. if statement
ii. if-else statement
iii. Switch statement.
i. if StatementThe basic form of the if statement is:
If (expression) statementIn this form, the expression is first evaluated. If the expression evaluates to non-zero (meaning TRUE), the statement is executed; if it evaluates to zero (meaning FALSE), the statement following the if statement is executed. Again, it has one entry point and one exit point.
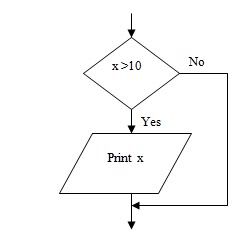
ii. if – else statementThe basic form of the if-else statement is :

In this form, the expression is first evaluated. If it evaluates to non-zero, statement_1 is executed, otherwise (i.e, if it evaluates to zero) statement_2 is executed. The execution of the statements are mutually exclusive, meaning, either statement_1 is executed or statement_2, but not both. The statement can, of course, take the form of blocks.
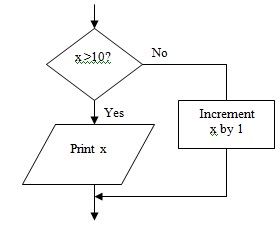
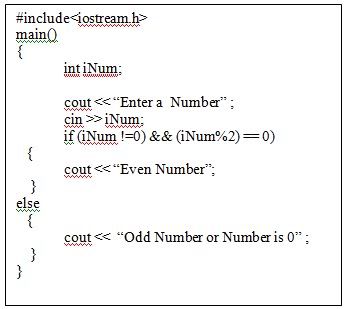
The if – else statement in the Example5.4 is to checks the value of iNum to be not equals to zero and also that the modulus value of the variable iNum is equal to zero. If condition is true, then the message ‘Even Number’ is printed on the screen. If condition is not true, then the message ‘Odd Number or Number is 0’ is printed on screen.
iii-Switch statementThe switch statement is the multiple branch decision statement is sometimes called the multiple-choice statement. The general form of the switch statement is :
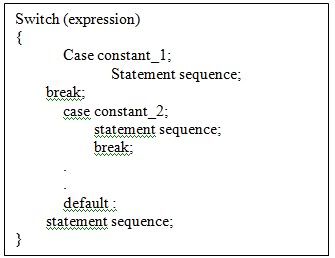
The expression evaluates to an integer or character constants and statement sequence is a block of statements.
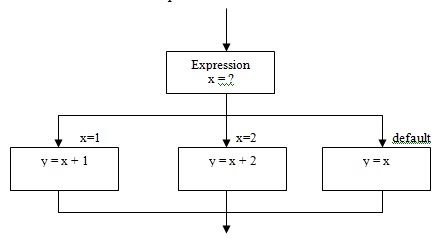
example:
The sample output of the program: Please key in grade (A-F) : C Minimun marks is 40In the example above, A,B,C and D are the possible values that can be assigned to the variable cGrade.
In each case of the constants, A to D, a statement or sequence of statements would be executed.
You would have noticed that every line has statement under it called “break”. The break is the only thing that stops a case statement from continuing all the way down through each case label under it. In fact, if you do not put break in, the program will keep going down in the case statements, into other case labels, until it reaches the end of a break.
However, the default part is the codes that would be executed if there were no matching case of constant’s values.